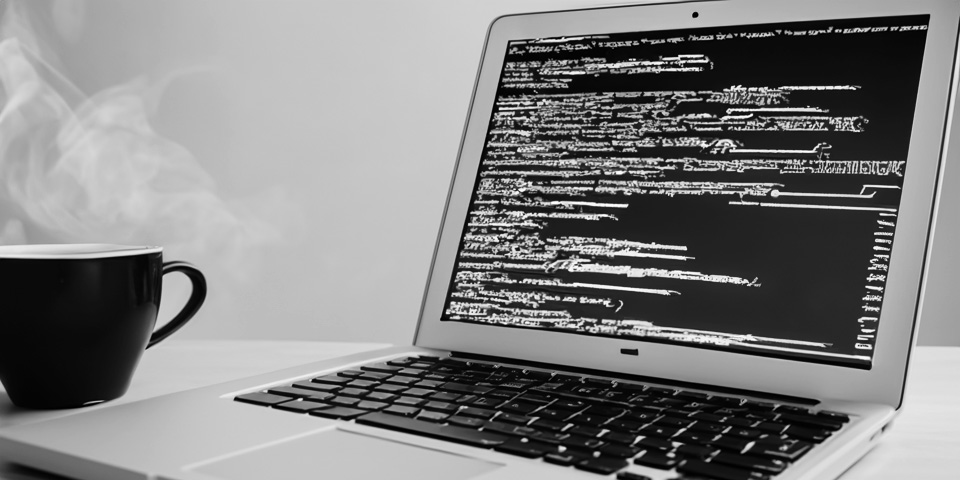
Angular2 UrlResolver for Component Assets
Thoughtram published a piece about Component Relative Paths in Angular2. This is great! It makes working with components much easier.
However, I wasn’t sure about other component-specific assets, like images for example. So I asked about it:
@dfbaskin That's a good point! There's no isolated solution for that yet
— Pascal Precht ʕ•̫͡•ʔ (@PascalPrecht) June 15, 2016
There is a solution, it turns out. There may be some better options in the future, but you can use the UrlResolver object to do this.
You can inject the resolver object into your component and then create a resolvePath
function that can be used by the view.
import {Component} from '@angular/core';
import {UrlResolver} from '@angular/compiler';
@Component({
moduleId: module.id,
selector: 'title-page',
styleUrls: ['title-page.component.css'],
templateUrl: 'title-page.component.html'
})
export class TitlePageComponent {
constructor(private urlResolver: UrlResolver) {
}
public resolvePath(path) {
return this.urlResolver.resolve(module.id, path);
}
}
In the view, just bind an image element to the resolvePath
function.
<img [src]="resolvePath('angular.svg')" />
As the Thoughtram article mentioned, this solution is tied to using the CommonJS format for modules. In other words, the module.id
property I’m using here as an input into the UrlResolver
object would not exist for other formats.
Updated Mar-19-2017
This technique is now obsolete. https://angular.io/docs/ts/latest/guide/change-log.html